TypeScript Types and Interfaces: A Comprehensive Guide
Learn how to effectively use TypeScript's type system to create more robust and maintainable applications.
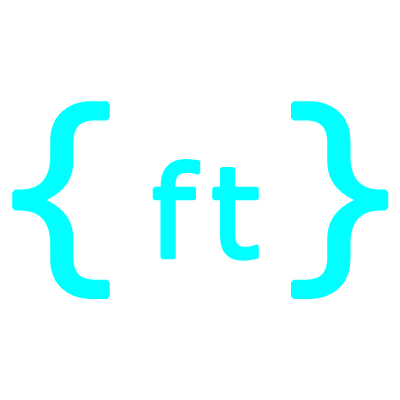
Francis Njenga
Lead Developer
TypeScript Types and Interfaces: A Comprehensive Guide
TypeScript has revolutionized JavaScript development by adding a powerful type system that helps catch errors during development rather than at runtime.
Article Highlights
- Core TypeScript types and their usage
- Difference between types and interfaces
- Advanced patterns with generics
- Type guards and narrowing techniques
- Best practices for type safety
Why Types Matter
JavaScript is dynamically typed, which means a variable's type can change during its lifetime. While this provides flexibility, it can lead to unexpected behavior and bugs that are difficult to trace.
TypeScript addresses this by adding static typing to JavaScript, allowing developers to define what types of values can be assigned to variables, passed to functions, and returned from functions.
Basic Types in TypeScript
TypeScript provides several basic types that will be familiar to JavaScript developers. These form the foundation of the type system.
Beyond the Basics: Advanced Types
TypeScript's type system goes far beyond simple primitives. Here are some powerful features:
- Union types for variables that can be one of several types
- Type aliases to create custom type names
- Literal types for exact value matching
- Nullable types using union with null/undefined
Interfaces: Defining Shapes
Interfaces are one of TypeScript's most powerful features. They allow you to define the structure that objects must conform to, including:
- Required and optional properties
- Readonly properties
- Method signatures
- Index signatures
Extending Interfaces
Interfaces can extend other interfaces, allowing for composition and reuse of type definitions. This is particularly useful for building complex type systems.
Types vs. Interfaces
Key Differences
- Interfaces can be extended with new properties later
- Types can use unions, conditional types, and other advanced features
- Interfaces are often preferred for public APIs
- Types are better for complex type operations
Function Types
TypeScript can also type functions, ensuring they receive and return the correct types. This includes:
- Parameter types
- Return types
- Optional parameters
- Rest parameters
- Function overloading
Generics: Reusable Components
Generics allow you to create reusable components that work with a variety of types while maintaining type safety. They're essential for building flexible yet type-safe libraries and utilities.
Type Guards and Type Narrowing
Type guards help TypeScript understand which type a variable is at a given point in your code, enabling more precise type checking and autocompletion.
Best Practices
TypeScript Type System Guidelines
- Prefer interfaces for public APIs
- Use explicit types for function parameters and return values
- Avoid using 'any' - prefer 'unknown' for type-safe alternatives
- Use generics for reusable code patterns
- Leverage union types for flexible yet type-safe variables
Conclusion
TypeScript's type system is a powerful tool for creating robust, maintainable code. By leveraging types and interfaces effectively, you can catch errors at compile time rather than runtime, making your applications more reliable and easier to refactor.
As your TypeScript skills advance, explore more advanced features like mapped types, conditional types, and the utility types provided by TypeScript to make your code even more expressive and type-safe.
// Basic types let isDone: boolean = false; let decimal: number = 6; let color: string = "blue"; let list: number[] = [1, 2, 3]; let tuple: [string, number] = ["hello", 10]; let x: any = "hello"; // any allows any type (use sparingly)
Basic TypeScript types demonstration
// Union types: variable can be one of several types let id: string | number; id = "abc123"; id = 123; // Both are valid // Type aliases: create a new name for a type type ID = string | number; let userId: ID = "user_123"; // Literal types: specific values that a variable can have type Direction = "north" | "south" | "east" | "west"; let userDirection: Direction = "north"; // userDirection = "northeast"; // Error! Type '"northeast"' is not assignable to type 'Direction'. // Nullable types let nullableString: string | null = "hello"; nullableString = null; // Valid with union type
Using advanced type patterns in TypeScript
interface User { id: number; name: string; email: string; age?: number; // Optional property (may or may not exist) readonly createdAt: Date; // Can't be modified after creation } function createUser(user: User): User { // Implementation return user; } const newUser: User = { id: 1, name: "John Doe", email: "john@example.com", createdAt: new Date() };
Using interfaces to define object shapes
interface BasicAddress { street: string; city: string; zipCode: string; } interface AddressWithCountry extends BasicAddress { country: string; } const address: AddressWithCountry = { street: "123 Main St", city: "Anytown", zipCode: "12345", country: "USA" };
Interface inheritance in TypeScript
// Using a type type Animal = { name: string; species: string; }; // Using an interface interface Animal { name: string; species: string; } // Extending a type (using intersection) type Pet = Animal & { owner: string; }; // Extending an interface interface Pet extends Animal { owner: string; }
Key differences between types and interfaces
// Function type type MathFunction = (a: number, b: number) => number; const add: MathFunction = (a, b) => a + b; const subtract: MathFunction = (a, b) => a - b; // Interface for function interface Calculator { (a: number, b: number): number; description?: string; } const multiply: Calculator = (a, b) => a * b; multiply.description = "Multiplication function";
Typing functions in TypeScript
// Generic function function identity<T>(arg: T): T { return arg; } const result = identity<string>("hello"); // Explicitly setting T to string const inferredResult = identity(42); // TypeScript infers T as number // Generic interface interface Box<T> { value: T; } const stringBox: Box<string> = { value: "hello" }; const numberBox: Box<number> = { value: 42 };
Using generics for type-safe, reusable components
function processValue(value: string | number) { // Type guard if (typeof value === "string") { // TypeScript knows value is a string here return value.toUpperCase(); } else { // TypeScript knows value is a number here return value.toFixed(2); } }
Using type guards for type narrowing
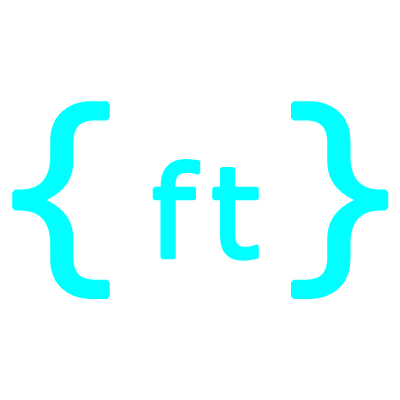
Francis Njenga
Lead Developer
Francis Njenga is an experienced Lead Developer specializing in React, Next.js, and modern JavaScript frameworks, with a strong background in web development.