Mastering Async/Await in Modern JavaScript
Learn how to use the async/await pattern to write cleaner, more maintainable asynchronous code in JavaScript.
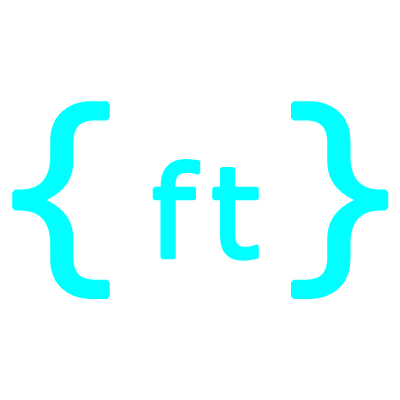
Francis Njenga
Lead Developer
Mastering Async/Await in JavaScript
Learn how to use the async/await pattern to write cleaner and more maintainable asynchronous code in JavaScript.
Article Summary
- Evolution from callbacks to promises to async/await
- How async/await makes code more readable
- Practical examples with comparison
- Best practices and common pitfalls
Understanding Asynchronous JavaScript
JavaScript is single-threaded, meaning it executes one task at a time. However, many tasks in web development are asynchronous:
- Network requests (API calls)
- File system operations
- Database queries
- Timers (setTimeout, setInterval)
- User interactions
The Evolution of Async Patterns
1. Callback Hell
The earliest approach used nested callbacks, leading to what's known as "callback hell" - deeply nested, hard-to-read code.
2. Promises
Introduced in ES6, promises provided better chaining and error handling through .then()
and
.catch()
.
3. Async/Await
The current standard (ES2017+) that makes asynchronous code look and behave like synchronous code, while maintaining non-blocking execution.
Best Practices
Do's and Don'ts
- DO use try/catch for error handling
- DO use Promise.all() for parallel operations
- DON'T forget await keywords
- DON'T use async/await in loops unnecessarily
getData(function(a) { getMoreData(a, function(b) { getEvenMoreData(b, function(c) { getTheFinalData(c, function(d) { // Do something with d }); }); }); });
This "callback hell" or "pyramid of doom" made debugging and error handling challenging.
getData() .then(a => getMoreData(a)) .then(b => getEvenMoreData(b)) .then(c => getTheFinalData(c)) .then(d => { // Do something with d }) .catch(error => { // Handle errors });
While better, chaining multiple .then() calls still didn't feel as natural as synchronous code.
Async/await simplifies asynchronous code by making it look and behave more like synchronous code.
async function processData() { try { const a = await getData(); const b = await getMoreData(a); const c = await getEvenMoreData(b); const d = await getTheFinalData(c); return d; } catch (error) { // Handle errors } }
Modern async/await pattern for cleaner asynchronous code
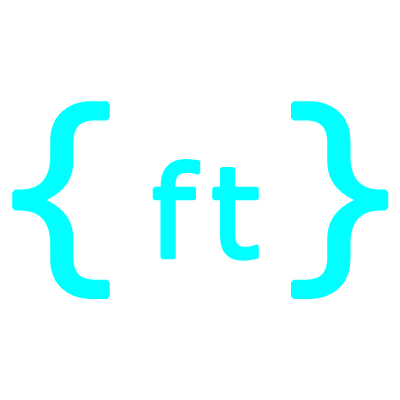
Francis Njenga
Lead Developer
Francis Njenga is an experienced Lead Developer specializing in React, Next.js, and modern JavaScript frameworks, with a strong background in web development.